🇹🇭 Thai Speech-to-Text (ASR) PRO 🆕
🇹🇭🆕 ระบบแปลงเสียงพูดภาษาไทยเป็นข้อความ (Pro Model)
Welcome to Thai ASR PRO - our advanced Thai Automatic Speech Recognition service powered by Large Language Models (LLMs). This version delivers superior accuracy and context-aware transcription while maintaining reasonable processing speed.
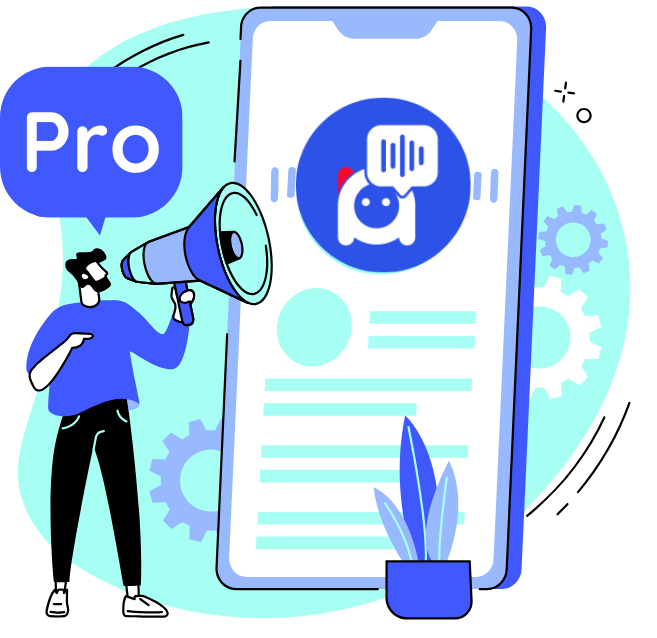
Visit our API Portal to test the Text to Speech API with your own voice.
Getting Started
-
Prerequisites
- An API key from iApp Technology
- Audio files in supported formats
- Supported formats: MP3, WAV, AAC, M4A
- Maximum file length: No more than 30 minutes
- Maximum file size: 1GB
-
Quick Start
- High accuracy transcription with context awareness
- Advanced LLM-powered processing
- Support for Thai language
-
Key Features
- Context-aware text extraction from audio files
- Enhanced accuracy for complex audio scenarios
- Speaker diarization
- Word-level timestamps
- Flexible JSON response format
-
Security & Compliance
- GDPR and PDPA compliant
- No data retention after processing
Please visit API Portal to view your existing API key or request a new one.
API Reference
Endpoint
POST
https://api.iapp.co.th/asr/v3
Headers
apikey
(required): Your API key for authentication- Additional headers are generated by FormData
Request Parameters
Parameter | Type | Description |
---|---|---|
file* | File (.mp3, .wav, .aac, .m4a) | Audio file to transcribe (No more than 30 minutes) |
chunk_size | Integer | Audio chunk size (recommended: 7) |
Code Examples
Python
import requests
url = "https://api.iapp.co.th/asr/v3"
payload = {'use_asr_pro': '1', 'chunk_size': '7'} #Set to '1' for iApp ASR PRO
files=[
('file',('{YOUR_UPLOADED_FILE}',open('{YOUR_UPLOADED_FILE_PATH}','rb'),'application/octet-stream'))
]
headers = {
'apikey': '{YOUR_API_KEY}'
}
response = requests.request("POST", url, headers=headers, data=payload, files=files)
print(response.text)
Javascript
const axios = require('axios');
const FormData = require('form-data');
const fs = require('fs');
let data = new FormData();
data.append('file', fs.createReadStream('YOUR_UPLOADED_FILE'));
data.append('use_asr_pro', '1'); // Set to '1' for iApp ASR PRO
data.append('chunk_size', '7');
let config = {
method: 'post',
maxBodyLength: Infinity,
url: 'https://api.iapp.co.th/asr/v3',
headers: {
'apikey': '{YOUR_API_KEY}',
...data.getHeaders()
},
data : data
};
axios.request(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
PHP
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.iapp.co.th/asr/v3',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS => array('file'=> new CURLFILE('{YOUR_UPLOADED_FILE}'),
'use_asr_pro' => '1',
'chunk_size' => '7'),
CURLOPT_HTTPHEADER => array(
'apikey: {YOUR_API_KEY}'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
Swift
let parameters = [
[
"key": "file",
"src": "{YOUR_UPLOADED_FILE}",
"type": "file"
],
[
"key": "use_asr_pro",
"value": "1",
"type": "text"
],
[
"key": "chunk_size",
"value": "7",
"type": "text"
]] as [[String: Any]]
let boundary = "Boundary-\(UUID().uuidString)"
var body = Data()
var error: Error? = nil
for param in parameters {
if param["disabled"] != nil { continue }
let paramName = param["key"]!
body += Data("--\(boundary)\r\n".utf8)
body += Data("Content-Disposition:form-data; name=\"\(paramName)\"".utf8)
if param["contentType"] != nil {
body += Data("\r\nContent-Type: \(param["contentType"] as! String)".utf8)
}
let paramType = param["type"] as! String
if paramType == "text" {
let paramValue = param["value"] as! String
body += Data("\r\n\r\n\(paramValue)\r\n".utf8)
} else {
let paramSrc = param["src"] as! String
let fileURL = URL(fileURLWithPath: paramSrc)
if let fileContent = try? Data(contentsOf: fileURL) {
body += Data("; filename=\"\(paramSrc)\"\r\n".utf8)
body += Data("Content-Type: \"content-type header\"\r\n".utf8)
body += Data("\r\n".utf8)
body += fileContent
body += Data("\r\n".utf8)
}
}
}
body += Data("--\(boundary)--\r\n".utf8);
let postData = body
var request = URLRequest(url: URL(string: "https://api.iapp.co.th/asr/v3")!,timeoutInterval: Double.infinity)
request.addValue("{YOUR_API_KEY}", forHTTPHeaderField: "apikey")
request.addValue("multipart/form-data; boundary=\(boundary)", forHTTPHeaderField: "Content-Type")
request.httpMethod = "POST"
request.httpBody = postData
let task = URLSession.shared.dataTask(with: request) { data, response, error in
guard let data = data else {
print(String(describing: error))
return
}
print(String(data: data, encoding: .utf8)!)
}
task.resume()
Kotlin
val client = OkHttpClient()
val mediaType = "text/plain".toMediaType()
val body = MultipartBody.Builder().setType(MultipartBody.FORM)
.addFormDataPart("file","{YOUR_UPLOADED_FILE}",
File("{YOUR_UPLOADED_FILE_PATH}").asRequestBody("application/octet-stream".toMediaType()))
.addFormDataPart("use_asr_pro","1")
.addFormDataPart("chunk_size","7")
.build()
val request = Request.Builder()
.url("https://api.iapp.co.th/asr/v3")
.post(body)
.addHeader("apikey", "{YOUR_API_KEY}")
.build()
val response = client.newCall(request).execute()
Java
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("text/plain");
RequestBody body = new MultipartBody.Builder().setType(MultipartBody.FORM)
.addFormDataPart("file","{YOUR_UPLOADED_FILE}",
RequestBody.create(MediaType.parse("application/octet-stream"),
new File("{YOUR_UPLOADED_FILE_PATH}")))
.addFormDataPart("use_asr_pro","1")
.addFormDataPart("chunk_size","7")
.build();
Request request = new Request.Builder()
.url("https://api.iapp.co.th/asr/v3")
.method("POST", body)
.addHeader("apikey", "{YOUR_API_KEY}")
.build();
Response response = client.newCall(request).execute();
Dart
var headers = {
'apikey': '{YOUR_API_KEY}'
};
var request = http.MultipartRequest('POST', Uri.parse('https://api.iapp.co.th/asr/v3'));
request.fields.addAll({
'use_asr_pro': '1',
'chunk_size': '7'
});
request.files.add(await http.MultipartFile.fromPath('file', '{YOUR_UPLOADED_FILE'));
request.headers.addAll(headers);
http.StreamedResponse response = await request.send();
if (response.statusCode == 200) {
print(await response.stream.bytesToString());
}
else {
print(response.reasonPhrase);
}
Accuracy and Performance
Overall Accuracy
Benchmark results on Thai Testset from Mozilla Common Voice dataset. We evaluated iApp ASR PRO performance across two different versions of the test set.
Test Conditions
- Unseen dataset
- Thai language only
- Speaker diversity: Male, Female, Children
Mozilla Common Voice 17.0 Thai Test Set
Visit the Dataset on Hugging Face
Results:
- Test Set Size: 11,042 samples
- Average Word Error Rate (WER) : 0.0801 or 8.01%
- Average Character Error Rate (CER) : 0.0219 or 2.19%
- Average Accuracy based on WER : 91.99%
- Average Accuracy based on CER : 97.81%
Processing Speed
- Optimized for accuracy over speed
Pricing
AI API Service Name | Endpoint | IC Per Seconds | On-Premise |
---|---|---|---|
Thai Speech To Text (ASR) | iapp-asr-v3-en [Pro Model] | 2 IC/60 Seconds | Contact |
iapp-asr-v3-th-en [Pro Model] | 2 IC/60 Seconds |