💳 Thai Credit Card Statement OCR 🆕
💳 ใบแจ้งยอดบัตรเครดิตไทย
Welcome to the Thai Credit Card Statement OCR API, an AI-powered solution developed by iApp Technology Co., Ltd. for extracting data from Thai credit card statements. This documentation will help you integrate and use our OCR service effectively.
Try Demo!
Example Images (Click to try)
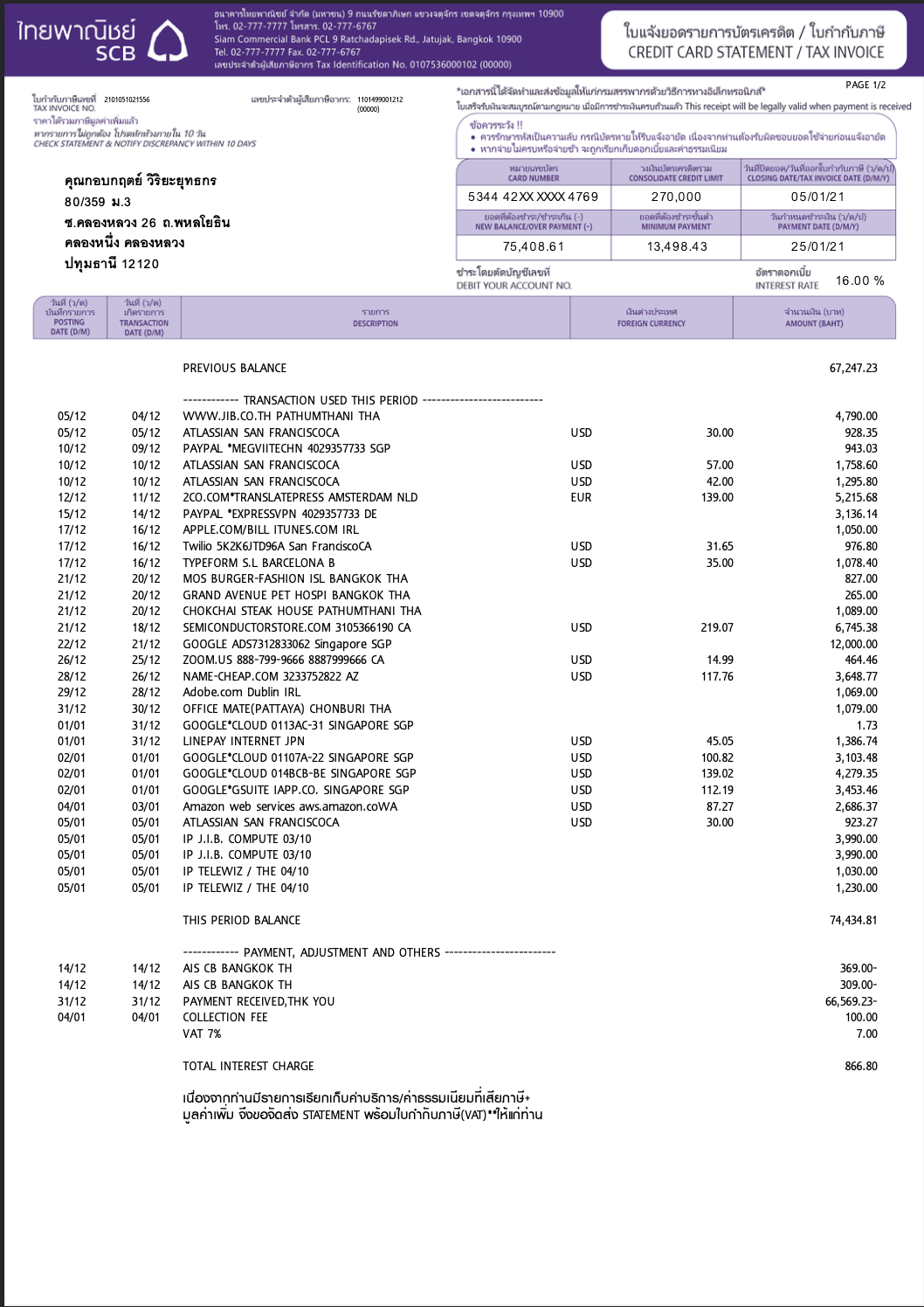
Demo key is limited to 10 requests per day per IP
Click here to get your API key
Getting Started
-
Prerequisites
- An API key from iApp Technology
- Thai credit card statement images
- Supported file formats: JPEG, JPG, PNG, HEIC, HEIF, PDF
- Maximum file size: 10MB
-
Quick Start
- Fast processing (5-10 seconds per document)
- High accuracy text extraction
- Support for multiple file formats
-
Key Features
- Detailed field extraction including:
- Statement details (type, dates, card number)
- Card holder information (name)
- Bank information (name, address, tax ID, contact)
- Transaction details (date, description, amount)
- Financial summary (balances, payments, charges)
- Reward points information
- Support for both single-page and multi-page documents (PDF)
- Option to return original OCR text and processed images
- Flexible JSON response format with detailed field extraction
- Detailed field extraction including:
-
Security & Compliance
- GDPR and PDPA compliant
- Secure API endpoints
- No data retention after processing
Example
Here's a sample credit card statement and its extracted data:
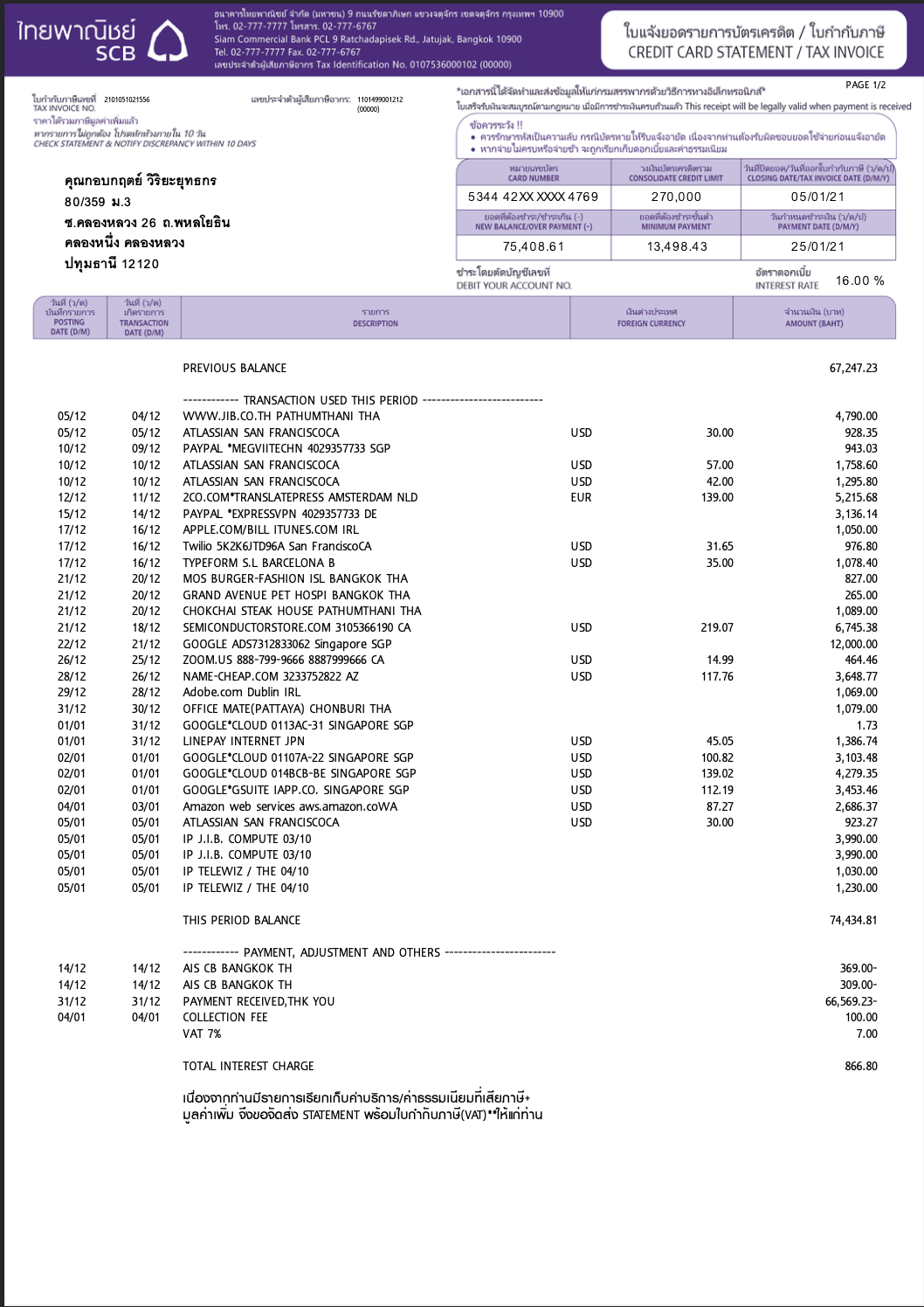
Request:
cURL
curl -X POST "https://api.iapp.co.th/ocr/v3/creditcard-statement/file" \
-H "apikey: YOUR_API_KEY" \
-F "file=@/path/to/creditcard-statement.jpg" \
-F "return_image=false" \
-F "return_ocr=false"
How to get API Key?
Please visit iApp AI Portal to view your existing API key or request a new one.
Response:
{
"message": "success",
"processed": {
"statementType": "ใบแจ้งยอดบัญชีบัตรเครดิต",
"statementDate": "31/12/2566",
"dueDate": "25/01/2567",
"cardNumber": "4711-XXXX-XXXX-1234",
"cardHolderName": "นาย ทดสอบ ระบบ",
"bankName": "ธนาคารกสิกรไทย จำกัด (มหาชน)",
"bankAddress": "1 ซอยราษฎร์บูรณะ 27/1 ถนนราษฎร์บูรณะ แขวงราษฎร์บูรณะ เขตราษฎร์บูรณะ กรุงเทพฯ 10140",
"bankTaxID": "0107536000315",
"bankPhone": "02-888-8888",
"bankFax": "02-888-8888",
"creditLimit": 100000.0,
"closingDate": "31/12/2566",
"newBalancePayment": 15789.4,
"minimumPaymentDue": 789.47,
"interestRate": 16.0,
"paymentByDebitAccountNo": "XXX-X-XX789-X",
"transactions": [
{
"transactionDate": "20/12",
"postingDate": "21/12",
"description": "CHOKCHAI STEAK HOUSE PATHUMTHANI THA",
"foreignAmount": null,
"foreignCurrencyCode": null,
"amountTHB": 1089.0
}
],
"payments_adjustment_others": [
{
"transactionDate": "31/12",
"postingDate": "31/12",
"description": "PAYMENT RECEIVED, THK YOU",
"foreignAmount": null,
"foreignCurrencyCode": null,
"amountTHB": -369.0
}
],
"previousBalance": 14789.4,
"thisPeriodBalance": 15789.4,
"totalPayments": 678.0,
"totalInterestCharges": 0.0,
"reward": {
"rewardAccumulatedPoints": 1234,
"rewardPointsEarned": 56,
"bonusPointsEarned": 0,
"pointsAdjustedThisMonth": 0,
"pointsRedeemed": 0,
"pointsOutstanding": 1290
},
"paymentBarcodeNo": "1234567890123456789"
},
"process_ms": 6821
}