👤 Face Detection
👤 ระบบตรวจจับใบหน้า
Welcome to Face Detection API, an AI product developed by iApp Technology Co., Ltd. Our API is designed to detect and extract faces from images with high accuracy and speed. The API supports both single and multiple face detection with flexible scoring options.
Try Demo!
Example Images (Click to try)
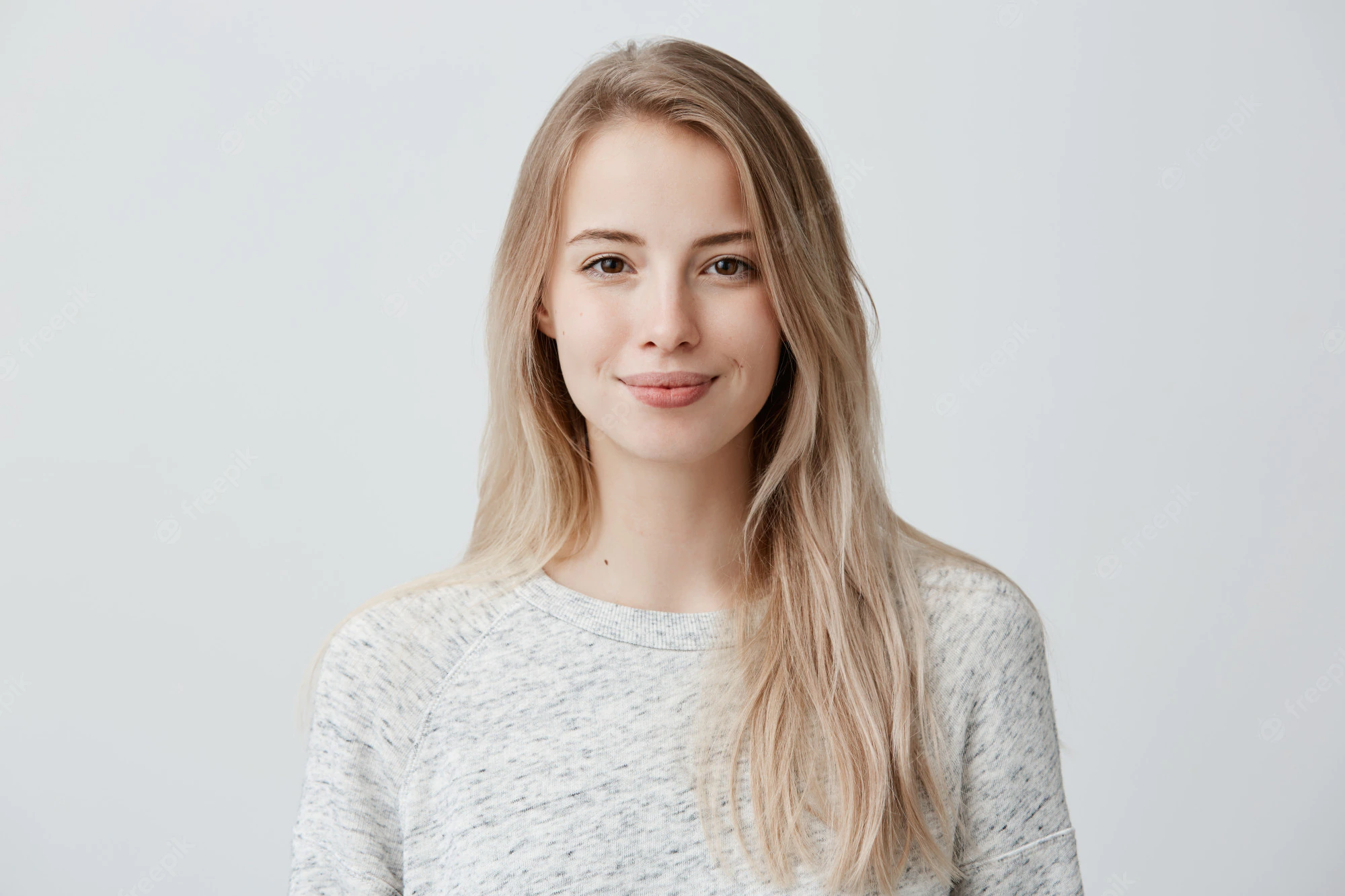
Demo key is limited to 10 requests per day per IP
Click here to get your API key
Getting Started
-
Prerequisites
- An API key from iApp Technology
- Image files containing faces
- Supported formats: JPEG, JPG, PNG
- Maximum file size: 10MB
-
Quick Start
- Fast processing time
- High accuracy face detection
- Support for multiple file formats
- Flexible scoring options
-
Key Features
- Single and multiple face detection
- Face bounding box coordinates
- Detection confidence scores
- Face image extraction
- Company-specific scoring options
-
Security & Compliance
- GDPR and PDPA compliant
- No data retention after processing
How to get API Key?
Please visit API Portal to view your existing API key or request a new one.
Example
Single Face Detection Request:
Image Preview
curl --location --request POST 'https://api.iapp.co.th/face_detect_single' \
--header 'apikey: {Your API Key}' \
--form 'file=@"{Your Image File Path}"'
Single Face Detection Response:
{
"bbox": {
"xmax": 320.15,
"xmin": 100.23,
"ymax": 450.78,
"ymin": 150.45
},
"detection_score": 0.9998989105224609,
"face": "/9j/4AAQ...base64_encoded_image",
"message": "success",
"process_time": 0.41
}
Multiple Face Detection Request:
Image Preview
curl --location --request POST 'https://api.iapp.co.th/face_detect_multi' \
--header 'apikey: {Your API Key}' \
--form 'file=@"{Your Image File Path}"'
Multiple Face Detection Response:
{
"message": "success",
"process_time": 0.67,
"result": [
{
"bbox": {
"xmax":1317.7054443359375,
"xmin":1075.64013671875,
"ymax":555.1968994140625,
"ymin":243.38815307617188
},
"detection_score": 0.99,
"face": "/9j/4AAQ...base64_encoded_image"
},
{
"bbox": {
"xmax":684.1102294921875,
"xmin":484.45068359375,
"ymax":520.4813842773438,
"ymin":265.50823974609375
},
"detection_score": 0.99,
"face": "/9j/4AAQ...base64_encoded_image"
},
...
]
}
Features & Capabilities
Core Features
- Single and multiple face detection
- Face bounding box coordinates
- Detection confidence scores
- Base64 encoded face images
- Company-specific scoring options
Supported Fields
Single Face Detection Response
Field | Type | Description |
---|---|---|
bbox | Dictionary | Face bounding box coordinates |
detection_score | Float | Face detection confidence score |
face | String | Base64 encoded face image |
message | String | Processing status |
process_time | Float | Processing time in seconds |
Multiple Face Detection Response
Field | Type | Description |
---|---|---|
message | String | Processing status |
process_time | Float | Processing time in seconds |
result | Array | Array of detected faces |
bbox | Dictionary | Face bounding box coordinates |
detection_score | Float | Face detection confidence score |
face | String | Base64 encoded face image |
Code Examples
Python
import requests
url = "https://api.iapp.co.th/face_detect_single"
payload = {}
files=[
('file',('{YOUR UPLOADED IMAGE}',open('{YOUR UPLOADED IMAGE}','rb'),'image/jpeg'))
]
headers = {
'apikey': '{YOUR API KEY}'
}
response = requests.request("POST", url, headers=headers, data=payload, files=files)
print(response.text)
JavaScript
const axios = require('axios');
const FormData = require('form-data');
const fs = require('fs');
let data = new FormData();
data.append('file', fs.createReadStream('/Users/iapp/Downloads/removebg_trial.png'));
let config = {
method: 'post',
maxBodyLength: Infinity,
url: 'https://api.iapp.co.th/face_detect_single',
headers: {
'apikey': 'YOUR_API_KEY',
...data.getHeaders()
},
data : data
};
axios.request(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
PHP
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.iapp.co.th/face_detect_single',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS => array('file'=> new CURLFILE('{YOUR UPLOADED IMAGE}')),
CURLOPT_HTTPHEADER => array(
'apikey: {YOUR API KEY}'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
Swift
let parameters = [[
"key": "file",
"src": "{YOUR UPLOADED IMAGE}",
"type": "file"
]] as [[String: Any]]
let boundary = "Boundary-\(UUID().uuidString)"
var body = Data()
var error: Error? = nil
for param in parameters {
if param["disabled"] != nil { continue }
let paramName = param["key"]!
body += Data("--\(boundary)\r\n".utf8)
body += Data("Content-Disposition:form-data; name=\"\(paramName)\"".utf8)
if param["contentType"] != nil {
body += Data("\r\nContent-Type: \(param["contentType"] as! String)".utf8)
}
let paramType = param["type"] as! String
if paramType == "text" {
let paramValue = param["value"] as! String
body += Data("\r\n\r\n\(paramValue)\r\n".utf8)
} else {
let paramSrc = param["src"] as! String
let fileURL = URL(fileURLWithPath: paramSrc)
if let fileContent = try? Data(contentsOf: fileURL) {
body += Data("; filename=\"\(paramSrc)\"\r\n".utf8)
body += Data("Content-Type: \"content-type header\"\r\n".utf8)
body += Data("\r\n".utf8)
body += fileContent
body += Data("\r\n".utf8)
}
}
}
body += Data("--\(boundary)--\r\n".utf8);
let postData = body
var request = URLRequest(url: URL(string: "https://api.iapp.co.th/face_detect_single")!,timeoutInterval: Double.infinity)
request.addValue("{YOUR API KEY}", forHTTPHeaderField: "apikey")
request.addValue("multipart/form-data; boundary=\(boundary)", forHTTPHeaderField: "Content-Type")
request.httpMethod = "POST"
request.httpBody = postData
let task = URLSession.shared.dataTask(with: request) { data, response, error in
guard let data = data else {
print(String(describing: error))
return
}
print(String(data: data, encoding: .utf8)!)
}
task.resume()
Kotlin
val client = OkHttpClient()
val mediaType = "text/plain".toMediaType()
val body = MultipartBody.Builder().setType(MultipartBody.FORM)
.addFormDataPart("file","file",
File("{YOUR API KEY}").asRequestBody("application/octet-stream".toMediaType()))
.build()
val request = Request.Builder()
.url("https://api.iapp.co.th/face_detect_single")
.post(body)
.addHeader("apikey", "{YOUR API KEY}")
.build()
val response = client.newCall(request).execute()
Java
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("text/plain");
RequestBody body = new MultipartBody.Builder().setType(MultipartBody.FORM)
.addFormDataPart("file","file",
RequestBody.create(MediaType.parse("application/octet-stream"),
new File("{YOUR UPLOADED IMAGE}")))
.build();
Request request = new Request.Builder()
.url("https://api.iapp.co.th/face_detect_single")
.method("POST", body)
.addHeader("apikey", "{YOUR API KEY}")
.build();
Response response = client.newCall(request).execute();
Dart
var headers = {
'apikey': '{YOUR API KEY}'
};
var request = http.MultipartRequest('POST', Uri.parse('https://api.iapp.co.th/face_detect_single'));
request.files.add(await http.MultipartFile.fromPath('file', '{YOUR UPLOADED IMAGE}'));
request.headers.addAll(headers);
http.StreamedResponse response = await request.send();
if (response.statusCode == 200) {
print(await response.stream.bytesToString());
}
else {
print(response.reasonPhrase);
}
Limitations and Best Practices
Limitations
- Maximum file size: 10MB
- Supported formats: JPEG, JPG, PNG
- Maximum number of faces per image: 50
Best Practices
- Ensure good image quality
- Check detection scores
- Handle error messages appropriately
- Consider using company-specific scoring
- Optimize image size before upload
Accuracy & Performance
Overall Accuracy
- Detection score typically above 95%
- Performance varies with image quality
Processing Speed
- Single face: 0.3-0.5 seconds
- Multiple faces: 0.5-1.0 seconds
Factors Affecting Accuracy
- Image Quality
- Resolution
- Lighting
- Focus
- Face Orientation
- Head pose
- Facial expressions
- Occlusions
History
Version 1.0 (Released: Jan 2023)
- Key Improvements: Initial Release
Version 0.9 (Released: Nov 2022)
- Key Improvements: Beta Testing
Version 0.8 (Released: Sep 2022)
- Key Improvements: Internal Testing
Pricing
AI API Service Name | Endpoint | IC Per Request | On-Premise |
---|---|---|---|
Face Detection | iapp_face_detection_v2_multiple_face | 0.2 IC/Request | Contact |
iapp_face_detection_v2_single_face | 0.2 IC/Request |