🚗 Thai Driver License OCR
🚗 ใ บขับขี่ไทย
Welcome to Thai Driver License OCR API, version 1.1, an AI product developed by iApp Technology Co., Ltd. Our API is designed to extract text information from the front face of Thai Driver License card images with high accuracy and speed. Our API supports JPEG, JPG, PNG file formats and can process a driver license image in 1-2 seconds.
Try Demo!
Example Images (Click to try)
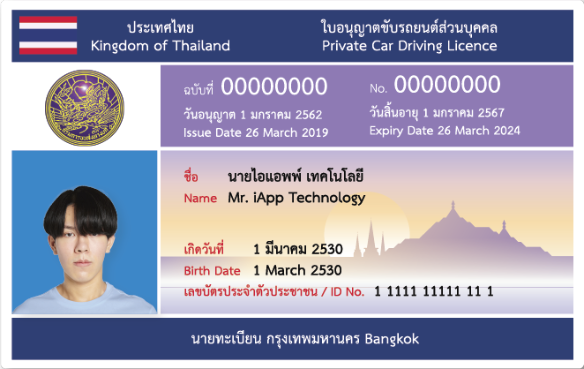
Demo key is limited to 10 requests per day per IP
Click here to get your API key
Getting Started
-
Prerequisites
- An API key from iApp Technology
- Thai driver license images (front)
- Supported file formats: JPEG, JPG, PNG
- Maximum file size: 10MB
-
Quick Start
- Fast processing (1-2 seconds per card)
- High accuracy text extraction (95.14% at character level)
- Support for multiple file formats
-
Key Features
- Detailed field extraction including:
- License number
- Full name (Thai and English)
- Date of birth
- Issue and expiry dates
- ID number
- Registrar information
- Support for front side of driver license
- Option to return face photo in base64 format
- Flexible JSON response format
- Detailed field extraction including:
-
Security & Compliance
- GDPR and PDPA compliant
- No data retention after processing
How to get API Key?
Please visit API Portal to view your existing API key or request a new one.
Example
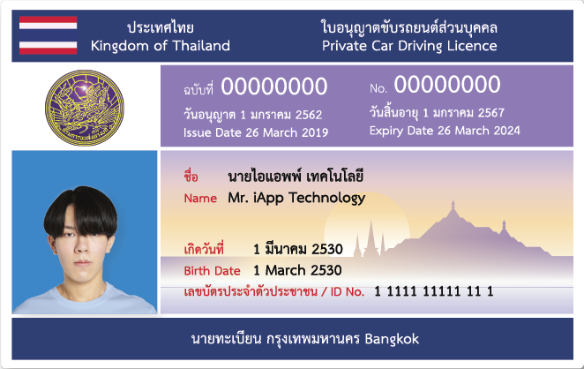
Request:
curl -X POST https://api.iapp.co.th/thai-driver-license-ocr \
-H "apikey: YOUR_API_KEY" \
-F "file=@/path/to/driver_license.jpg"
Response:
{
"driving_license_type2": "Detected",
"driving_license_type6": "Detected",
"en_country": "Kingdom of Thailand",
"en_dob": "1 March 1987",
"en_expiry": "26 March 2024",
"en_issue": "18 May 2006",
"en_license_no": "00000000",
"en_name": "Mr. iApp Technology",
"en_type": "Private Car Driving License",
"face": "Detected",
"file_name": "thai-driver-license-ocr-example.png",
"flag": "Detected",
"id_no": "1111111111111",
"inference": "0.523",
"message": "Success",
"registrar": "นายทะเบียนจังหวัด กรุงเทพมหานคร Bangkok",
"sign": "Detected",
"status_code": 200,
"text_is_white": "Detected",
"th_country": "ประเทศไทย",
"th_dob": "1 มีนาคม 2530",
"th_expiry": "26 มีนาคม 2567",
"th_issue": "1 มกราคม 2562",
"th_license_no": "000000000",
"th_name": "นาย ไอแอพพ์ เทคโนโลยี",
"th_type": "ใบอนุญาติขับรถยนต์ส่วนบุคค ล"
}
Features & Capabilities
Core Features
- High accuracy text extraction (95.14% character-level accuracy)
- Fast processing time (1-2 seconds per card)
- Support for multiple file formats (JPEG, JPG, PNG)
- Automatic card detection
- Optional face photo extraction in base64 format
- Support for both Thai and English text extraction
Supported Fields
Field | Type | Description |
---|---|---|
en_country | String | Country name in English |
en_expiry | String | Expiry date in English |
en_issue | String | Issue date in English |
en_name | String | Full name in English |
en_license_no | String | License number in English |
en_type | String | License type in English |
face | String | Face detection status |
id_no | String | ID card number |
th_country | String | Country name in Thai |
th_expiry | String | Expiry date in Thai |
th_issue | String | Issue date in Thai |
th_name | String | Full name in Thai |
th_license_no | String | License number in Thai |
th_type | String | License type in Thai |
registrar | String | Registrar information |
API Reference
Recognizing Thai Driver License
This endpoint processes Thai driver license images and returns the extracted data in a structured format.
POST https://api.iapp.co.th/thai-driver-license-ocr
Request Headers
Name | Type | Required | Description |
---|---|---|---|
apikey | string | Yes | Your API key |
Request Body (multipart/form-data)
Parameter | Type | Required | Description |
---|---|---|---|
file | File | Yes | The binary data of the driver license image |
fields | String | No | Optional fields (e.g., "photo" for face photo) |
Code Examples
Python
import requests
url = "https://api.iapp.co.th/thai-driver-license-ocr"
payload = {
'fields': 'photo'
}
files = [
('file',('driver_license.jpg',open('path/to/driver_license.jpg','rb'),'image/jpeg'))
]
headers = {
'apikey': 'YOUR_API_KEY'
}
response = requests.request("POST", url, headers=headers, data=payload, files=files)
print(response.json())
JavaScript
const axios = require("axios")
const FormData = require("form-data")
const fs = require("fs")
const form = new FormData()
form.append("file", fs.createReadStream("path/to/driver_license.jpg"))
form.append("fields", "photo")
const config = {
method: "post",
url: "https://api.iapp.co.th/thai-driver-license-ocr",
headers: {
apikey: "YOUR_API_KEY",
...form.getHeaders(),
},
data: form,
}
axios(config)
.then((response) => console.log(JSON.stringify(response.data)))
.catch((error) => console.log(error))
PHP
<?php
$curl = curl_init();
$post_data = array(
'file'=> new CURLFile('path/to/driver_license.jpg'),
'fields' => 'photo'
);
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.iapp.co.th/thai-driver-license-ocr',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_POST => true,
CURLOPT_POSTFIELDS => $post_data,
CURLOPT_HTTPHEADER => array(
'apikey: YOUR_API_KEY'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
?>
Swift
import Foundation
let headers = [
"apikey": "YOUR_API_KEY"
]
let parameters = [
[
"name": "file",
"fileName": "path/to/driver_license.jpg"
],
[
"name": "fields",
"value": "photo"
]
]
let boundary = "Boundary-\(UUID().uuidString)"
var body = ""
var error: Error? = nil
for param in parameters {
if let fileName = param["fileName"] {
let fileContent = try? String(contentsOfFile: fileName, encoding: .utf8)
body += "--\(boundary)\r\n"
body += "Content-Disposition:form-data; name=\"\(param["name"] ?? "")\""
body += "; filename=\"\(fileName)\"\r\n"
body += "Content-Type: image/jpeg\r\n\r\n"
body += fileContent ?? ""
} else {
body += "--\(boundary)\r\n"
body += "Content-Disposition:form-data; name=\"\(param["name"] ?? "")\"\r\n\r\n"
body += param["value"] ?? ""
}
}
let postData = body.data(using: .utf8)
var request = URLRequest(url: URL(string: "https://api.iapp.co.th/thai-driver-license-ocr")!,timeoutInterval: Double.infinity)
request.addValue("YOUR_API_KEY", forHTTPHeaderField: "apikey")
request.httpMethod = "POST"
request.httpBody = postData
let task = URLSession.shared.dataTask(with: request) { data, response, error in
guard let data = data else {
print(String(describing: error))
return
}
print(String(data: data, encoding: .utf8)!)
}
task.resume()
Kotlin
import okhttp3.*
import java.io.File
val client = OkHttpClient()
val mediaType = MediaType.parse("image/jpeg")
val file = File("path/to/driver_license.jpg")
val body = MultipartBody.Builder().setType(MultipartBody.FORM)
.addFormDataPart("file", file.name, RequestBody.create(mediaType, file))
.addFormDataPart("fields", "photo")
.build()
val request = Request.Builder()
.url("https://api.iapp.co.th/thai-driver-license-ocr")
.addHeader("apikey", "YOUR_API_KEY")
.post(body)
.build()
client.newCall(request).execute().use { response ->
println(response.body()?.string())
}
Java
import java.io.File;
import okhttp3.*;
public class Main {
public static void main(String[] args) {
OkHttpClient client = new OkHttpClient().newBuilder().build();
MediaType mediaType = MediaType.parse("image/jpeg");
RequestBody body = new MultipartBody.Builder().setType(MultipartBody.FORM)
.addFormDataPart("file", "driver_license.jpg",
RequestBody.create(mediaType, new File("path/to/driver_license.jpg")))
.addFormDataPart("fields", "photo")
.build();
Request request = new Request.Builder()
.url("https://api.iapp.co.th/thai-driver-license-ocr")
.method("POST", body)
.addHeader("apikey", "YOUR_API_KEY")
.build();
try {
Response response = client.newCall(request).execute();
System.out.println(response.body().string());
} catch(Exception e) {
e.printStackTrace();
}
}
}
Dart
import 'package:http/http.dart' as http;
void main() async {
var request = http.MultipartRequest('POST',
Uri.parse('https://api.iapp.co.th/thai-driver-license-ocr'));
request.files.add(await http.MultipartFile.fromPath(
'file', 'path/to/driver_license.jpg'));
request.fields['fields'] = 'photo';
request.headers.addAll({
'apikey': 'YOUR_API_KEY'
});
http.StreamedResponse response = await request.send();
if (response.statusCode == 200) {
print(await response.stream.bytesToString());
} else {
print(response.reasonPhrase);
}
}
Limitations and Best Practices
Limitations
- Maximum file size: 10MB
- Supported formats: JPEG, JPG, PNG
- Front side only
Best Practices
- Ensure good image quality
- Check inference score in response
- Handle error messages appropriately
- Validate license number format
- Consider using fields parameter for specific needs
Accuracy & Performance
Overall Accuracy
- Character-level accuracy: 95.14%
- Field-specific accuracies:
- Face detection: 100%
- License number: 97.37%
- English name: 99.13%
- Thai name: 95.62%
Processing Speed
- Average: 1-2 seconds
- May vary based on image size and quality
Factors Affecting Accuracy
- Image Quality
- Resolution
- Lighting
- Focus
- Card Condition
- Physical damage
- Reflections
- Text clarity
History
Version | Release Date | Accuracy | Key Improvements |
---|---|---|---|
1.1 | Jan 2023 | 95.14% | Added face photo extraction, Increased accuracy |
1.0 | Dec 2022 | 90% | Initial Release |
Pricing
AI API Service Name | Endpoint | IC Per Request | On-Premise |
---|---|---|---|
Thai Driver License Card OCR [v1.1] | iapp_thai_driver_license_ocr | 1.25 IC/Request | Contact |