👤🔍 Face Recognition
👤🔍 ระ บบจดจำใบหน้า
Welcome to Face Recognition API, an AI product developed by iApp Technology Co., Ltd. Our API is designed to recognize faces in images with high accuracy and speed. The API supports both single and multiple face recognition with flexible scoring options.
Try Demo!
Example Images (Click to try)
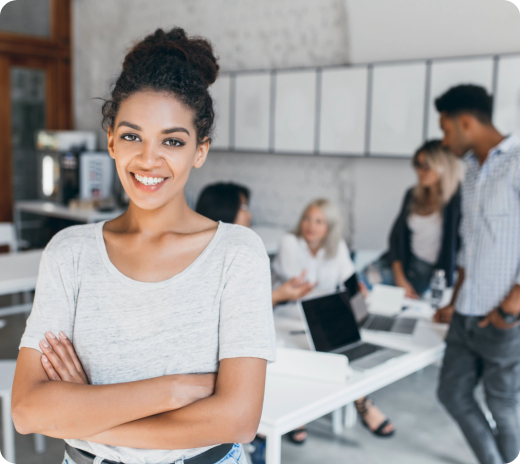
Demo key is limited to 10 requests per day per IP
Click here to get your API key
Getting Started
-
Prerequisites
- An API key from iApp Technology
- Image files containing faces
- Supported formats: JPEG, JPG, PNG
- Maximum file size: 2MB
-
Quick Start
- Fast processing time
- High accuracy face recognition
- Support for multiple file formats
- Flexible scoring options
-
Key Features
- Single and multiple face recognition
- Face bounding box coordinates
- Recognition confidence scores
- Face image extraction
- Company-specific scoring options
-
Security & Compliance
- GDPR and PDPA compliant
- No data retention after processing
Please visit API Portal to view your existing API key or request a new one.
Example
Face Recognition Request:
Image Preview
curl --location 'https://api.iapp.co.th/face_recog_single' \
--header 'apikey: {YOUR API KEY}' \
--form 'file=@"{YOUR UPLOADED FILE}"' \
--form 'company="{YOUR COMPANY NAME}"'
Face Recognition Response:
{
"bbox": {
"xmax": 255.44717407226562,
"xmin": 154.39295959472656,
"ymax": 282.8843688964844,
"ymin": 155.49319458007812
},
"company": "{YOUR COMPANY NAME}",
"detection_score": 0.9998624324798584,
"message": "successfully performed",
"name": "unknown",
"process_time": 0.4776473045349121,
"recognition_score": 0.0
}
Features & Capabilities
Core Features
- Single and multiple face recognition in images
- Face detection with bounding box coordinates
- Face feature extraction and matching
- Customizable detection and recognition score thresholds
- Face database management (add/remove faces)
- Export/import of face features
Supported Fields
- Face bounding box coordinates (xmin, xmax, ymin, ymax)
- Detection confidence score
- Recognition confidence score
- Person name identification
- Processing time
API Reference
Face Recognition Endpoints
-
Single Face Recognition (
/face_recog_single
)- Recognizes the most prominent face in an image
- Returns face position and identity
-
Multiple Face Recognition (
/face_recog_multi
)- Recognizes all faces in an image
- Returns array of face position and identities
-
Single Face Recognition (
/face_recog_facecrop
)- Recognizes the name of the person in the picture by using only face image
- Returns face position and identity
Code Examples
Python
import requests
def recognize_face(image_path, api_key, company):
url = "https://api.iapp.co.th/face_recog_single"
payload = {'company': {YOUR COMPANY NAME}}
files = [
('file', ({YOUR UPLOADED FILE}, open({YOUR UPLOADED FILE}, 'rb'), 'image/jpeg'))
]
headers = {
'apikey': {YOUR API KEY}
}
response = requests.post(url, headers=headers, data=payload, files=files)
return response.json()
JavaScript
const myHeaders = new Headers();
myHeaders.append("apikey", "{YOUR API KEY}");
const formdata = new FormData();
formdata.append("file", fileInput.files[0], "{YOUR UPLOADED FILE}");
formdata.append("company", "{YOUR COMPANY NAME}");
const requestOptions = {
method: "POST",
headers: myHeaders,
body: formdata,
redirect: "follow"
};
fetch("https://api.iapp.co.th/face_recog_single", requestOptions)
.then((response) => response.text())
.then((result) => console.log(result))
.catch((error) => console.error(error));
PHP
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.iapp.co.th/face_recog_single',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS => array(
'file' => new CURLFILE('{YOUR UPLOADED FILE}'),
'company' => '{YOUR COMPANY NAME}'
),
CURLOPT_HTTPHEADER => array(
'apikey: {YOUR API KEY}'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
Swift
let parameters = [
[
"key": "file",
"src": "{YOUR UPLOADED FILE}",
"type": "file"
],
[
"key": "company",
"value": "{YOUR COMPANY NAME}",
"type": "text"
]] as [[String: Any]]
let boundary = "Boundary-\(UUID().uuidString)"
var body = Data()
var error: Error? = nil
for param in parameters {
if param["disabled"] != nil { continue }
let paramName = param["key"]!
body += Data("--\(boundary)\r\n".utf8)
body += Data("Content-Disposition:form-data; name=\"\(paramName)\"".utf8)
if param["contentType"] != nil {
body += Data("\r\nContent-Type: \(param["contentType"] as! String)".utf8)
}
let paramType = param["type"] as! String
if paramType == "text" {
let paramValue = param["value"] as! String
body += Data("\r\n\r\n\(paramValue)\r\n".utf8)
} else {
let paramSrc = param["src"] as! String
let fileURL = URL(fileURLWithPath: paramSrc)
if let fileContent = try? Data(contentsOf: fileURL) {
body += Data("; filename=\"\(paramSrc)\"\r\n".utf8)
body += Data("Content-Type: \"content-type header\"\r\n".utf8)
body += Data("\r\n".utf8)
body += fileContent
body += Data("\r\n".utf8)
}
}
}
body += Data("--\(boundary)--\r\n".utf8);
let postData = body
var request = URLRequest(url: URL(string: "https://api.iapp.co.th/face_recog_single")!,timeoutInterval: Double.infinity)
request.addValue("{YOUR API KEY}", forHTTPHeaderField: "apikey")
request.addValue("multipart/form-data; boundary=\(boundary)", forHTTPHeaderField: "Content-Type")
request.httpMethod = "POST"
request.httpBody = postData
let task = URLSession.shared.dataTask(with: request) { data, response, error in
guard let data = data else {
print(String(describing: error))
return
}
print(String(data: data, encoding: .utf8)!)
}
task.resume()
Kotlin
val client = OkHttpClient()
val mediaType = "text/plain".toMediaType()
val body = MultipartBody.Builder().setType(MultipartBody.FORM)
.addFormDataPart("file","{YOUR UPLOADED FILE}",
File("{YOUR UPLOADED FILE}").asRequestBody("application/octet-stream".toMediaType()))
.addFormDataPart("company","{YOUR COMPANY NAME}")
.build()
val request = Request.Builder()
.url("https://api.iapp.co.th/face_recog_single")
.post(body)
.addHeader("apikey", "{YOUR API KEY}")
.build()
val response = client.newCall(request).execute()
Java
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("text/plain");
RequestBody body = new MultipartBody.Builder().setType(MultipartBody.FORM)
.addFormDataPart("file","{YOUR UPLOADED FILE}",
RequestBody.create(MediaType.parse("application/octet-stream"),
new File("{YOUR UPLOADED FILE}")))
.addFormDataPart("company","{YOUR COMPANY NAME}")
.build();
Request request = new Request.Builder()
.url("https://api.iapp.co.th/face_recog_single")
.method("POST", body)
.addHeader("apikey", "{YOUR API KEY}")
.build();
Response response = client.newCall(request).execute();
Dart
var headers = {
'apikey': '{YOUR API KEY}'
};
var request = http.MultipartRequest('POST', Uri.parse('https://api.iapp.co.th/face_recog_single'));
request.fields.addAll({
'company': '{YOUR COMPANY NAME}'
});
request.files.add(await http.MultipartFile.fromPath('file', '{YOUR UPLOADED FILE}'));
request.headers.addAll(headers);
http.StreamedResponse response = await request.send();
if (response.statusCode == 200) {
print(await response.stream.bytesToString());
}
else {
print(response.reasonPhrase);
}
Limitations and Best Practices
Limitations
- Maximum image size: 2MB
- Minimum image dimensions: 600x400 pixels
- Minimum face size: 112x112 pixels
- Supported formats: JPG, JPEG, PNG
- Queue length restrictions apply
Best Practices
- Use well-lit, clear face images
- Ensure faces are not obscured
- Submit appropriate image sizes
- Handle API errors appropriately
- Set optimal detection/recognition thresholds
Accuracy & Performance
Overall Accuracy
- High accuracy for frontal faces
- Performance varies with image quality
- Recognition accuracy depends on registered face quality
Processing Speed
- Typical response time: 0.3-1.0 seconds
- May vary with server load
- Queue system manages high traffic
Factors Affecting Accuracy
- Image quality and lighting
- Face angle and expression
- Face size in image
- Number of registered faces
- Detection/recognition threshold settings
History
The Face Recognition System has evolved to provide robust face detection and recognition capabilities while maintaining high performance and accuracy standards.
Pricing
AI API Service Name | Endpoint | IC Per Request | On-Premise |
---|---|---|---|
Face Recognition | iapp_face_recog_v2_multiple_face | 0.5 IC/Request | Contact |
iapp_face_recog_v2_only_face | 0.3 IC/Request | ||
iapp_face_recog_v2_single_face | 0.3 IC/Request |