ðĪðŠŠ āļāļēāļĢāļāļĢāļ§āļāļŠāļāļāđāļāļŦāļāđāļēāđāļĨ āļ°āļāļąāļāļĢāļāļĢāļ°āļāļēāļāļāļŠāļģāļŦāļĢāļąāļ KYC
ðĪðŠŠ AI āđāļāļĢāļĩāļĒāļāđāļāļĩāļĒāļāđāļāļŦāļāđāļēāļāļēāļāļĢāļđāļ Selfie āđāļĨāļ° āļĢāļđāļāļāļąāļāļĢāļāļĢāļ°āļāļēāļāļ āļŠāļģāļŦāļĢāļąāļāļāļēāļ KYC
āđāļĒāļĩāđāļĒāļĄāļāļĄ API Portal āļāļāļāđāļĢāļēāđāļāļ·āđāļāļāļāļŠāļāļ API āļāļēāļĢāļāļĢāļ§āļāļŠāļāļāđāļāļŦāļāđāļēāđāļĨāļ°āļāļąāļāļĢāļāļĢāļ°āļāļēāļāļāļŠāļģāļŦāļĢāļąāļ KYC āļāđāļ§āļĒāļĢāļđāļāļ āļēāļāļāļāļāļāļļāļāđāļāļ
āļāļāļĨāļāļāđāļāđāđāļĨāļĒ!â
āļĢāļđāļ āļ āļēāļāļāļąāļ§āļāļĒāđāļēāļāļāļąāļ§āđāļāļ (āļāļĨāļīāļāđāļāļ·āđāļāļĨāļāļ)
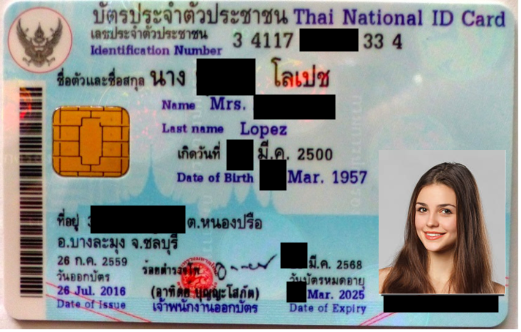
āļĢāļđāļāļ āļēāļāļāļąāļ§āļāļĒāđāļēāļāļāļąāļāļĢāļāļĢāļ°āļāļēāļāļ (āļāļĨāļīāļāđāļāļ·āđāļāļĨāļāļ)
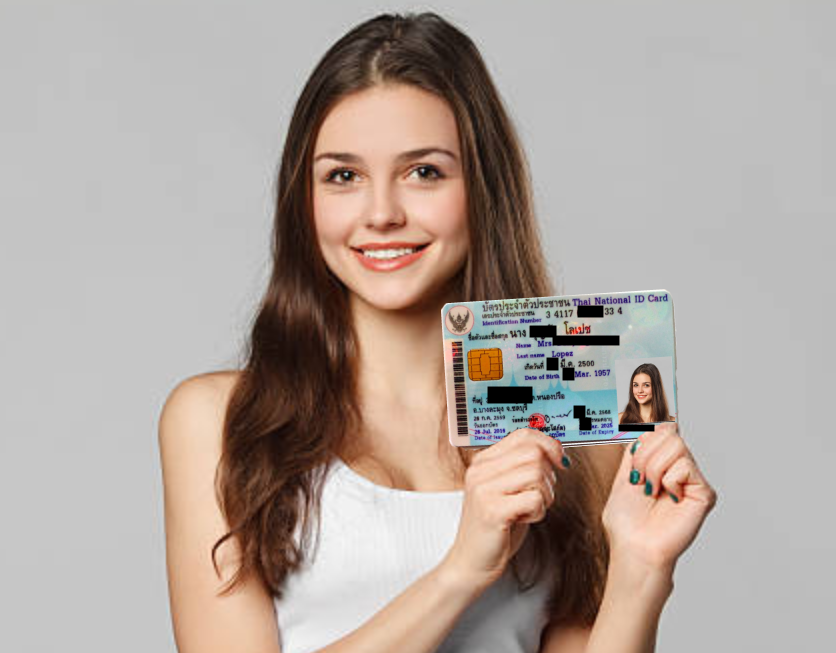
Demo key is limited to 10 requests per day per IP
Click here to get your API key
āđāļĢāļīāđāļĄāļāđāļāđāļāđāļāļēāļâ
āļāļēāļĢāļāļĢāļ§āļāļŠāļāļāđāļāļŦāļāđāļēāđāļĨāļ°āļāļąāļāļĢāļāļĢāļ°āļāļēāļāļāļŠāļģāļŦāļĢāļąāļ KYC āļāļ·āļāļāļĢāļīāļāļēāļĢāļāļĩāđāđāļāđāļāļĨāļąāļāļāļāļ AI āđāļāļāļēāļĢāđāļāļĢāļĩāļĒāļāđāļāļĩāļĒāļāļĢāļđāļāļāđāļēāļĒāđāļāļĨāļāļĩāđāļāļąāļāļĢāļđāļāļāđāļēāļĒāļāļąāļāļĢāļāļĢāļ°āļāļēāļāļāđāļāļ·āđāļāļĒāļ·āļāļĒāļąāļāļāļąāļ§āļāļāļŠāļģāļŦāļĢāļąāļāļ§āļąāļāļāļļāļāļĢāļ°āļŠāļāļāđ KYC (Know Your Customer)
āļāļąāļ§āļāļĒāđāļēāļâ
āļāļĢāļīāļāļēāļĢāļāļĩāđāđāļāđāļāđāļāļĄāļđāļĨāļāđāļāļāđāļāđāļēāļŠāļāļāļāļĒāđāļēāļ:
- āļĢāļđāļāļ āļēāļāļāļąāļāļĢāļāļĢāļ°āļāļēāļāļ (āļĢāļđāļāļ āļēāļāļāļāļēāļāđāļŦāļāđ)
- āļĢāļđāļāļ āļēāļāđāļāļĨāļāļĩāđāļāļāļāļāļđāđāļāļ·āļāļāļąāļāļĢāļāļĢāļ°āļāļēāļāļ (āļĢāļđāļāļ āļēāļāļāļāļēāļāđāļĨāđāļ)
āļāļēāļāļāļąāđāļāļāļ°āļ§āļīāđāļāļĢāļēāļ°āļŦāđāđāļĨāļ°āļāļĢāļ§āļāļŠāļāļāļāļ§āļēāļĄāļāļđāļāļāđāļāļāļ§āđāļēāļĢāļđāļāļ āļēāļāļāļąāđāļāļŠāļāļāđāļŠāļāļāļāļļāļāļāļĨāđāļāļĩāļĒāļ§āļāļąāļāļŦāļĢāļ·āļāđāļĄāđ